Python Testing with Pytest: The Basics and a Quick Tutorial
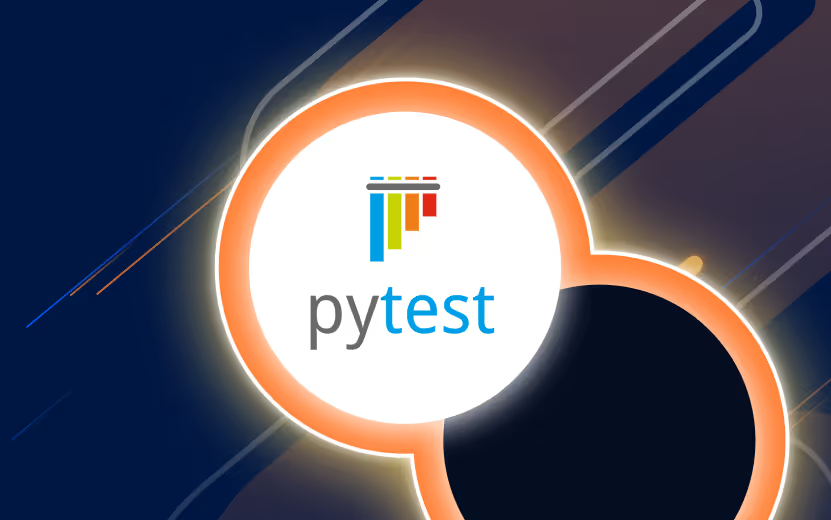
Pytest is a testing framework for Python that makes it easy to write small, scalable tests. It offers a new approach to unit testing, which is less complicated and more fun. Pytest is not only used for testing; it can also be used as a development aid. You can use it to write functional tests, integration tests, and even complex functional tests.
Pytest promotes a simple and easy-to-understand testing style. You can write test cases as functions instead of having to use classes. This way, you can start with simple test functions, and as your tests grow more complex, you can scale up to classes, modules, and packages.
Pytest also provides flexibility by allowing the use of third-party plugins. There are over 1,000 plugins available, and you can write your own, so if you need a feature that is not available in the core Pytest you can easily extend it with plugins.
This is part of a series of articles about API Testing
Unlike other testing frameworks, Pytest doesn't require a steep learning curve. You don't need to remember complex syntax or rules. All you need is your test code and some assertions, and Pytest takes care of the rest.
The simplicity of Pytest makes it easy to write tests, encouraging more developers to write tests for their code. This leads to better-maintained code and fewer bugs in production. Pytest's simplicity doesn't mean it's limited in functionality; it's a powerful framework that can handle all Python testing needs.
Managing state and dependencies is a critical part of testing. Pytest provides a clear and concise way to manage state and dependencies through fixtures. Fixtures are functions that Pytest will run before your test functions. They're used to set up some state or objects that your tests will use.
This approach makes your tests cleaner and easier to read. It eliminates the need for setup and teardown methods that can clutter your test code. Your tests become more modular and more maintainable. Fixtures also provide a powerful way to manage dependencies. You can use them to mock objects, inject dependencies, or even control the execution of your tests. This makes your tests more robust and reliable.
Pytest provides an easy way to run a subset of your tests. This is particularly useful when you have a large test suite and want to run a specific set of tests. Pytest allows you to filter tests based on their name, the name of the file they're in, or even their location in the file.
This feature saves time, and also makes it easier to integrate your tests into your development workflow. You can run a specific set of tests after each change, ensuring that your changes don't break any existing functionality.
Related content: Read our guide to api testing types
Another powerful feature of Pytest is test parameterization. It allows you to run a test function multiple times with different parameters. This is useful for testing functions that take different arguments.
Test parameterization can dramatically reduce the amount of test code you need to write. Instead of writing multiple tests for different cases, you can write a single test and run it with different parameters. This also makes your tests easier to read and maintain.
Pytest has a plugin-based architecture. This means you can extend its functionality with plugins. There are hundreds of plugins available for Pytest, and you can even write your own if you need a feature that's not available.
This plugin-based architecture makes Pytest incredibly flexible. You can tailor it to fit your exact needs. Whether you need to integrate with a specific tool, add a new kind of assertion, or even change how Pytest runs your tests, there's likely a plugin for that.
Related content: Read our guide to api test automation
Application Programming Interfaces (APIs) are becoming a big part of software development. Chances are that if you use Pytest, at some point you will use it to test an API. Here are three ways Pytest makes API testing easier.
Related content: Read our guide to api test automation
Fixtures in Pytest offer a way to manage test setup and teardown efficiently. They allow the configuration of necessary states or environments, like authentication processes or database connections.
For example, a fixture can establish an authenticated session for an API, which can then be utilized across multiple test functions. This approach streamlines the testing process by reducing code duplication and improving test isolation and performance.
Parameterization allows the execution of the same test across various input parameters, which is essential for APIs that handle diverse input. By utilizing the @pytest.mark.parametrize decorator, testers can create versatile test functions. For instance, a test for a GET request can be parameterized to test different query parameters, enhancing the coverage and efficiency of the tests.
Custom assertions are particularly useful in API testing to encapsulate the validation logic of API responses. For instance, a custom assertion can be created to verify response status codes, simplifying the test code and improving error reporting.
Additionally, Pytest's plugin ecosystem further extends its capabilities in API testing. Plugins like pytest-bdd for behavior-driven development and pytest-httpx for efficient HTTP client functionality can be seamlessly integrated, offering a tailored API testing experience.
Pytest and Unittest are probably the two most popular testing frameworks in Python. Both are powerful tools in a developer's arsenal, but they have distinct characteristics and use cases.
Unittest, Python's standard unit testing module, is known for its rich and robust features. It's the default testing framework in Python and follows the xUnit architecture. This framework is excellent for complex testing needs due to its wide range of assertion methods and setup/teardown methods for tests.
Pytest is known for its simplicity and ease of use. It's a no-boilerplate testing framework, meaning it does not require you to write as much code as Unittest does for a similar set of tests. Pytest also supports the features available in Unittest and nose (a now-defunct project that extended the capabilities of Unittest), making it as powerful as its alternative.
The primary difference between Pytest and Unittest lies in their design philosophies. While Unittest follows an object-oriented approach requiring you to create a class for each test case, Pytest favors a more straightforward function-based approach. Pytest also uniquely supports fixtures, which simplify setup and teardown processes in complex test scenarios.
Pytest is compatible with Python 2.6 and 3.4+. Thus, if you are using an older version of Python, you may need to upgrade.
To install Pytest, you can use pip, Python's package installer. Open your command line or terminal and type the following command:
After successful installation, you can verify it by running the command:
This should return the current version of Pytest installed on your system. Now that we have Pytest installed let's move on to creating our first test.
First, let's create a simple Python function. Let's name our file math_operations.py and define a function addition(). Here's how our code will look like:
Now, we will create a test for this function. In Pytest, test files should start or end with test, so Pytest can identify them. Create a new file named test_math_operations.py, and let's write our first test:
In this code, we import our addition function from the math_operations module. We then define a test function test_addition(). In this function, we assert that our addition function returns the correct output.
Store the above code as p1.py, and then execute it using this command: pytest p1.py
Assertions play a vital role in Pytest. They allow us to set conditions that we expect our code to meet. When the assertion condition is not met, Pytest provides detailed reports to help identify the problem.
Consider the previous example; the assert keyword checks whether the output of the addition function equals 5. If it does not, the test will fail, and Pytest will provide an informative error message.
Pytest supports various types of assertions such as equality, comparison, and checking for truthiness. You can also use assert with Python's built-in data structures like lists and dictionaries.
Running tests in parallel can significantly shorten the total testing time, especially when dealing with large test suites. Pytest allows us to run tests in parallel using the pytest-xdist plugin.
To install the pytest-xdist plugin, run the following command:
After successfully installing pytest-xdist, you can use the -n option followed by the number of CPUs you want to use for running the tests. For example, to use 4 CPUs:
This command will distribute the test execution across 4 CPUs, effectively reducing the testing time.
Parameterizing test cases is a powerful feature offered by Pytest. It allows us to run a test function multiple times with different arguments, leading to more efficient and cleaner test code.
Consider our previous test_addition function. Suppose we want to test the function with different sets of numbers. We can do this using Pytest's @pytest.mark.parametrize decorator. Here's how:
In this code, we parametrize the test function with three sets of numbers. The test_addition function runs three times, each time with a different pair of numbers and expected output.