Rest Assured: Java API Testing Made Easy (with Tutorial)
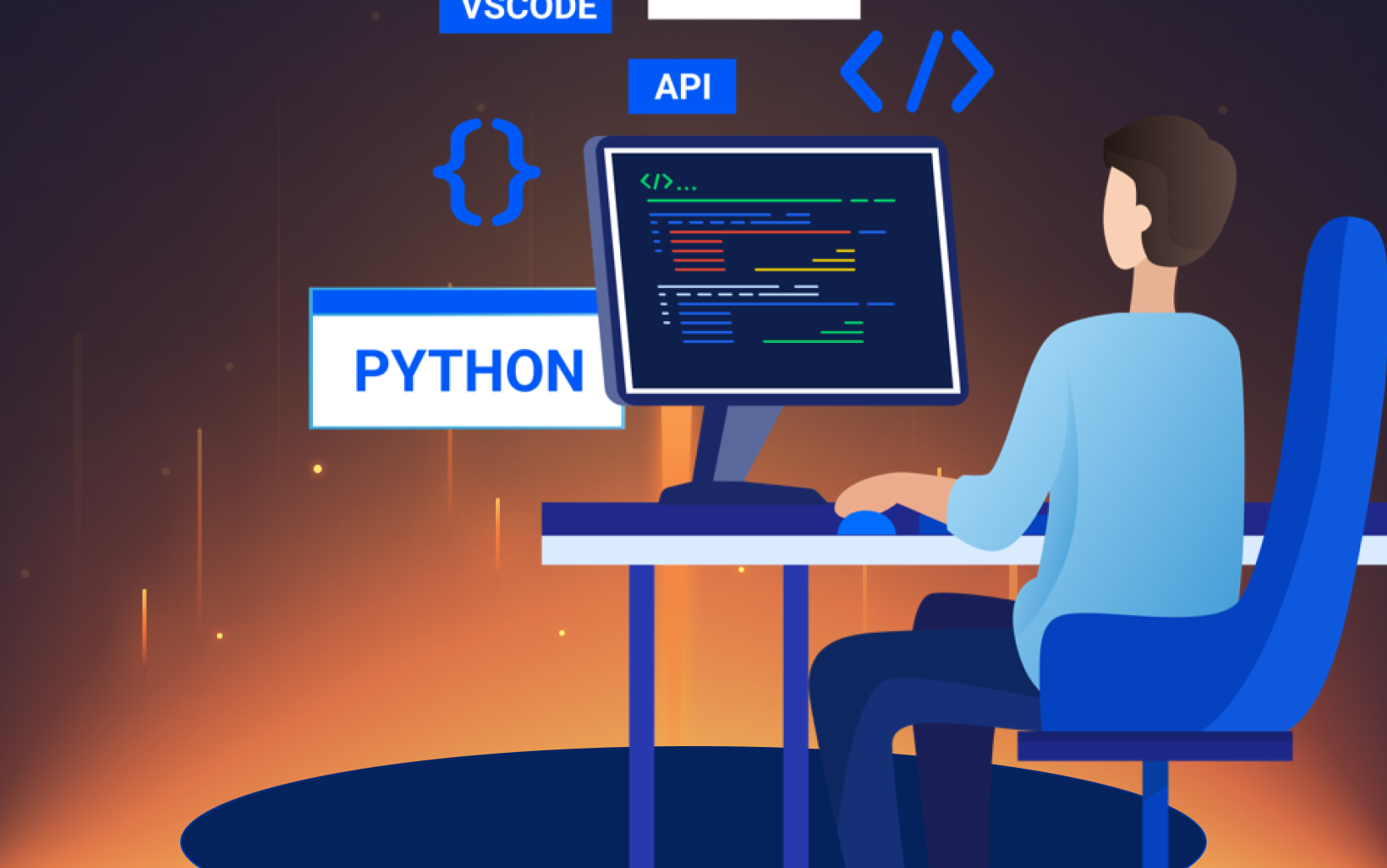
Rest Assured is an open-source framework for testing and validating REST APIs in Java, which allows you to write comprehensive tests with minimal code. It simplifies the testing process, making the Java API testing experience closer to that of dynamic languages like Ruby and Groovy.
Rest Assured supports a variety of HTTP methods, including GET, POST, PUT, DELETE, OPTIONS, PATCH, and HEAD. It also supports validations and can be integrated with Serenity, a behavioral driven development (BDD) automation framework.
Rest Assured provides a domain-specific language (DSL) that lets you write powerful, readable tests for your RESTful APIs. This DSL includes a wide variety of commands and assertions that you can use to validate your API’s response and status code. You can also validate headers, body, and cookies.
You can download Rest Assured from its official GitHub repo.
This is part of a series of articles about API testing
Rest Assured offers a straightforward and efficient way to test and validate your REST APIs. This is crucial in ensuring that your APIs perform as expected, provide the correct output, and handle errors gracefully.
Rest Assured provides everything you need to write effective tests, including a user-friendly DSL, support for different HTTP methods, and validations. Rest Assured also supports BDD Given/When/Then syntax, which makes your tests more readable and understandable.
Moreover, Rest Assured is open source, which means it's continually evolving and improving. You can contribute to its development, and you can benefit from the contributions of other developers.
Let’s jump into a tutorial showing how to set up and use the basic functionality of REST Assured.
Rest Assured is a library, not a standalone tool, which means it needs to be integrated into your project. For Maven projects, include the following dependencies in your pom.xml:
For Gradle projects, include the following in your build.gradle:
These dependencies will add Rest Assured to your project, and you will be able to import it into your test classes.
Once you have included Rest Assured in your project, you can write your first test. Let's assume we have a simple API that returns a greeting message.
In the code above, we use the given(), when(), get(), then(), and assertThat() methods provided by Rest Assured. The given() method sets up the test, the when().get() makes a GET request to the /greeting endpoint, and the then().assertThat() verifies the response.
Rest Assured provides several ways to validate the technical response data from the API. It includes methods to check the status code, headers, and content type, among others.
Validating status code
Validating the status code is one of the most basic checks you can perform on the response. It indicates whether the request was successful or not. A status code of 200 means the request was successful, while a 404 means the requested resource was not found.
In the test above, we check if the status code of the response is 200, which means the request was successful.
Validating headers
Headers provide additional information about the response or request. With Rest Assured, we can easily check the value of a specific header.
In the test above, we check if the Content-Type header of the response is application/json.
Validating content type
The content type is another important aspect of the response. It tells the client what the content of the response is, and how to parse it. Rest Assured allows us to check the content type of the response.
In the test above, we check if the content type of the response is html/text.
Parameterizing tests allows you to run the same test with different inputs. It is an essential practice in testing, as it allows you to cover more ground with less code.
In the test above, we pass different values for input and expected to the test. The testSquare() method is run five times, each time with a different pair of input and expected values.
Sometimes, you may want to pass parameters between tests, for example, when you want to create a resource in one test, and delete it in another. Rest Assured provides several ways to do this.
In the test above, we create a new resource with the post() method and extract the ID from the response with the extract().path() method. We then pass this ID as a path parameter to the delete() method to delete the resource.
Rest Assured also provides support for accessing secured APIs. It supports multiple authentication mechanisms, including Basic, Digest, and OAuth.
Basic Authentication
In Basic Authentication, the client sends the user name and password as part of the request header. Rest Assured provides the auth() method to easily set up Basic Authentication.
In the test above, we use the auth().basic() method to set up Basic Authentication with the user name "username" and password "password".
OAuth Authentication
OAuth is a more complex authentication mechanism that provides clients with "secure delegated access" to server resources on behalf of a resource owner. Rest Assured supports OAuth 1.0a, OAuth 2.0, and OAuth 2.0 with Bearer Access Token.
In the test above, we use the auth().oauth2() method to set up OAuth 2.0 authentication with the access token "accessToken".