API Testing: Definition, Examples, Tools, and Best Practices
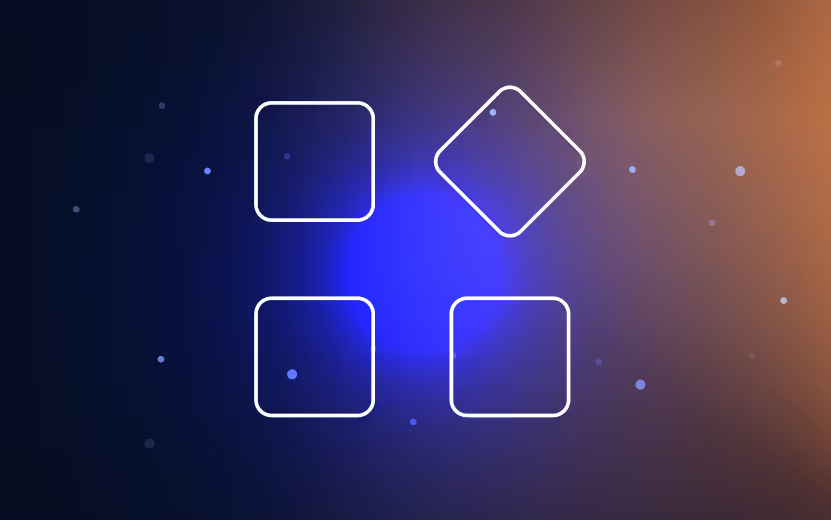
API testing is a type of software testing that involves testing APIs, either directly or as part of integration testing, to determine if they meet the expectations for functionality, reliability, performance, and security. Application programming interfaces (APIs) specify how software components should interact and allow different software systems to communicate with each other.
The goal of API testing is to ensure that the software systems communicate as expected. Typically, this is achieved by sending a request to the API and getting a response. The response is then assessed to determine if the API behaves as expected.
Most types of API testing are black box tests, where the tester doesn’t need to know the internal structure of the APIs. However some types of API testing, such as unit testing, are white box tests that examine the source code and structure or the application.
This is part of an extensive series of guides about DevOps.
API testing is a crucial part of the software development process for several reasons. First, it ensures that a software system that exposes or uses APIs works correctly. APIs are commonly used for critical functions of a software program, or might be an interface provided directly to users of the program.
API testing is also vital for ensuring the performance and reliability of a software system. If an API can't handle the load or isn't reliable, it could cause the software system to crash or behave unpredictably. This could have serious consequences, especially for systems that handle sensitive data or are used in critical operations.
Finally, API testing is essential for ensuring the security of the software. APIs are a common target for hackers, who can exploit vulnerabilities to gain unauthorized access to the system. By testing the API, developers can identify and fix these vulnerabilities before they are exploited.
There are several types of API tests that developers can perform to ensure the quality of their APIs. Here are some examples:
Validation testing is a type of API testing that ensures that the API is functioning correctly and providing the expected output. This usually involves sending a request to the API and comparing the response to the expected output.
For example, if an API is designed to provide weather data, a validation test might involve sending a request for the weather in a specific location and checking that the response includes the correct data.
Functional testing focuses on the business requirements of the API. It involves testing the API's functionality to ensure it performs its intended tasks correctly.
For example, a functional test for a payment API might involve sending a request to make a payment and checking that the payment is processed correctly.
Load testing checks how the API handles a large amount of traffic. This involves sending a large number of requests to the API and monitoring its performance.
For example, a load test for a streaming API might involve sending requests to stream a video from multiple devices simultaneously and checking that the API can handle the load without crashing or slowing down.
Reliability testing checks how the API performs over a long period. This involves sending requests to the API over a prolonged period and monitoring its performance.
For example, a reliability test for a data storage API might involve sending requests to store and retrieve data over several weeks and checking that the API performs consistently.
Security testing checks for vulnerabilities in the API. This involves sending requests that attempt to exploit potential vulnerabilities and monitoring the API's response.
For example, a security test for a user authentication API might involve sending requests with incorrect credentials and checking that the API doesn't provide unauthorized access.
Penetration testing, often referred to as pentesting, is a type of security testing used to uncover vulnerabilities, threats, risks in a software application that an attacker could exploit. The purpose of this test is to secure sensitive data from outsiders like hackers.
In the context of API testing, penetration testing might involve testing the API endpoints for possible security vulnerabilities. For example, a penetration test might attempt to bypass authorization to access secure data, or exploit vulnerabilities in the API to cause denial of service.
Fuzz testing or 'fuzzing' is a type of testing where large amounts of random data, called fuzz, are inputted into a system to uncover issues such as crashes, failing built-in code, or memory leaks.
In API testing, fuzzing could involve sending random, unexpected, or invalid data to the API endpoints to observe how they handle it. For instance, if an API expects a JSON object and a fuzz test sends invalid JSON syntax, or an XML document, the API should handle this gracefully, returning an appropriate error message and not crashing or exposing sensitive data.
Unit testing for API code focuses on testing individual components of the API in isolation. This involves verifying the functionality of specific functions or methods within the API without considering their interactions with other parts of the system. Unit tests are typically written and executed by developers as they write the API code, ensuring each unit functions correctly before integrating it into the larger system.
For instance, if an API has a function to calculate tax based on a given amount, a unit test would validate this function by providing different amounts and checking if the tax calculation is correct. This type of testing is crucial for identifying and fixing bugs early in the development process. Effective unit testing of APIs often involves mock objects and stubs to simulate the behavior of complex external systems or dependencies.
Integration testing is a level of software testing where individual units are integrated and tested as a group. For APIs, this could involve testing a sequence of API calls to ensure they work together correctly.
For example, an eCommerce application might have separate API endpoints for adding an item to the cart, updating the quantity of the item, and checking out. An integration test would ensure that these APIs work correctly together, that the correct sequence of calls results in the expected outcome.
Like any other testing activity, API testing comes with its fair share of challenges. Let's explore some of these.
APIs are often complex, with numerous endpoints, each possibly accepting a variety of input parameters and returning a variety of output formats. Without comprehensive, accurate, and up-to-date documentation, understanding how to test these APIs effectively can be difficult.
For example, if an API endpoint accepts a JSON object, the documentation should clearly specify the structure of this object, the type and range of permissible values for each field, and the expected behavior of the endpoint for various input values.
Addressing edge cases in API testing involves identifying and testing scenarios that are outside of normal operating parameters but still within the scope of the API's functionality. Edge cases often include unusual or extreme input values, unexpected user behaviors, or scenarios where multiple API features interact in complex ways. Testing for these cases is crucial because they can lead to unexpected behaviors or failures in the API, which might not be uncovered by standard testing approaches.
For example, an edge case in an API that processes user input might involve very long strings, special characters, or unexpected data types. Ensuring the API can handle these inputs correctly, either by processing them appropriately or by returning a meaningful error message, is essential. Addressing edge cases helps improve the robustness and reliability of the API, ensuring it behaves predictably under a wide range of conditions.
APIs often act as the interface between different software systems. Ensuring that these systems can interact with the API seamlessly and as expected can be a challenging task. For example, if an API is designed to accept XML data, it must ensure that it can handle XML data from different systems, which might format the data a bit differently.
The complexity of the request and response body in API testing can pose a significant challenge. The data sent to and received from API endpoints can often be complex, multi-level JSON or XML documents. Parsing these documents to extract the necessary information for testing can make API testing more difficult. However, API testing tools can help extract API responses and make it easily readable to developers.
Postman is an API platform designed to enhance the entire API lifecycle, from development to deployment, making it simpler and faster to create APIs. It supports various stages of the API lifecycle, including design, testing, documentation, and monitoring.
Key features:
Apigee, part of Google Cloud, is an API management platform designed to assist in building, managing, and securing APIs. It provides high-performance API proxies that create a consistent interface for backend services, offering control over aspects like security, rate limiting, quotas, and analytics.
Key features:
Source: Google Cloud
Cypress is a front-end testing tool for web applications, used for creating, debugging, and automatically running tests within continuous integration (CI) builds. Its straightforward installation process and API make it a popular choice for developers looking to test end-to-end functionality, and UI components directly in the browser.
Key features:
REST-assured is an open-source Java library that streamlines the testing process of RESTful APIs. It focuses on validating and interacting with RESTful web services. It offers features for making HTTP requests, validating responses, and performing detailed assertions on the data. It is compatible with Java-based test frameworks.
Key features:
Learn more in our detailed guide to api testing tools
There are several measures that can be taken to ensure the effectiveness of API tests.
When conducting effective API testing, it is important to accurately specify the API output status. This involves defining what the expected outcome of the API test should be, which serves as a benchmark against which the actual test result can be compared.
Identifying the output status helps in understanding whether the API is functional and behaving as expected. For instance, if the API is supposed to retrieve data from a database, the expected output status could be the data in a specific format. The actual output can then be compared with the expected output to check if the API is working correctly.
When it comes to API testing, it's always more efficient to focus on small functional APIs. Testing smaller APIs, which perform specific functions, is easier and more manageable than testing larger, more complex APIs.
With this approach, you can isolate each function and thoroughly test its performance. It allows for more detailed testing and helps in identifying any functional issues at an early stage. It also makes it easier to pinpoint the source of any problems that may arise during the testing process.
Of course, this approach assumes that the API design decomposes complex APIs into smaller functional APIs. A design using large, monolithic APIs is typically harder to test.
Organize API Endpoints
API endpoints refer to the points of interaction where APIs can exchange data or perform some function. Organizing these endpoints can help in maintaining a clear structure of the API and its various components.
One effective way to organize API endpoints is by grouping them based on their functionality. For instance, all endpoints related to user management (such as user creation, deletion, and modification) can be grouped together. This approach simplifies the testing process as you can focus on testing each group of endpoints as a unit.
Related content: Read our guide to pytest
Another useful practice is to document the API endpoints. This includes details such as the endpoint's URL, the method (GET, POST, etc.), and the expected input and output. Having a well-documented list of API endpoints can be a valuable reference during the testing process.
Positive tests are designed to confirm that the API works as expected under normal conditions. These tests usually involve inputting valid data and checking if the API returns the expected output.
Negative tests are designed to check how the API handles invalid data or unexpected conditions. These tests are essential in determining the robustness of the API and its ability to handle errors gracefully.
Together, positive and negative tests ensure a comprehensive evaluation of the API's functionality and reliability.
Learn more in our detailed guide to api testing types
Leveraging automation for API testing can help you save time, reduce manual effort, and improve the overall quality of your testing process.
Automated API testing tools can execute a large number of tests in a short time, thereby increasing the test coverage. These tools can also generate detailed reports, which can help you analyze the test results more effectively.
However, while automation is beneficial, manual testing still plays a vital role in the testing process. Therefore, the best approach is to strike a balance between automation and manual testing to ensure a comprehensive and effective API testing process.
Related content: Read our guide to api test automation
Security testing is a critical component of API testing, aimed at identifying and mitigating potential security vulnerabilities. APIs often handle sensitive data and are a common target for cyberattacks, making their security a top priority. Security testing involves various practices such as validating encryption methodologies, verifying authentication and authorization mechanisms, and ensuring that confidential data is handled securely.
One effective approach is to simulate potential security breaches to see how the API reacts. Dynamic testing tools can identify common vulnerabilities like SQL injection, Cross-Site Scripting (XSS), and Cross-Site Request Forgery (CSRF). Additionally, it is crucial to check for any exposed sensitive data like API keys, personal information.
Learn more about Pynt for API security testing
Together with our content partners, we have authored in-depth guides on several other topics that can also be useful as you explore the world of DevOps.
Authored by Anodot
Authored by Codefresh
Authored by Finout